Playwright Visual Regression Testing
Playwright Test supports visual comparisons, where it will take a screenshot of a page and then compare it with a 'golden image'. A golden image is a snapshot of what the correct visual layout should be.
Once you have a snapshot, your test can check if the layout still matches the one saved in the snapshot. If it does not match, your test will fail.
To get started, let's create a test called playwright_test.spec.js
with the following contents:
const { test, expect } = require('@playwright/test')
test('should add an item', async ({ page }) => {
await page.goto('https://testingbot.com')
const title = await page.title()
await base.expect(page).toHaveScreenshot()
})
Next, make sure you've created a playwright.config.js configuration file, which contains details such as:
- How to connect to the TestingBot browser grid
- On which remote browsers this visual test should run
To start the test, please run this command:
The first run will fail, because there's no 'golden image' yet.
When you run the test again, Playwright will take a new screenshot and compare it with the golden snapshot.
You can configure the threshold, maxDiffPixels
to your liking:
Masked Screenshots
Playwright provides the option to mask any specific locator when taking a screenshot.
This is useful if you want to do regression testing, and you know that a specific part of the page has content that might cause the test to fail or is not important for your test.
For example an ad, random list, or anything else that should not impact your visual comparison test.
Simply find one or more locators of elements you want to hide:
Finally, take a screenshot but mask the locators:
Below is an example of a screenshot with a masked locator:
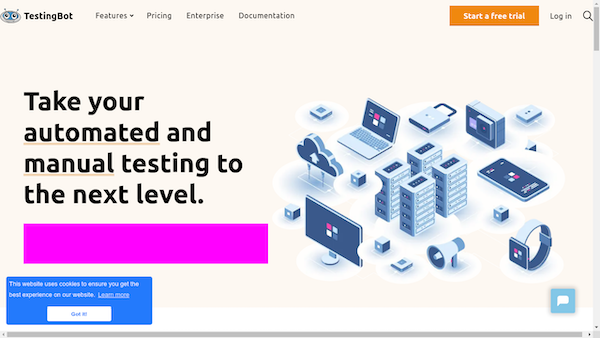
Text and binary snapshots
Next to comparing images, Playwright also supports comparing text or other (binary) data, through snapshots.
The example below shows how to compare the text of a DOM element to make sure it matches its snapshot.