PHP Automated Testing
- You can download PHP for Windows from https://windows.php.net/download/.
- Run the installer and follow the setup wizard to install PHP.
- Make sure you can use PHP with your command line: https://www.php.net/manual/en/install.windows.php.
PHP should already be present on macOS by default.
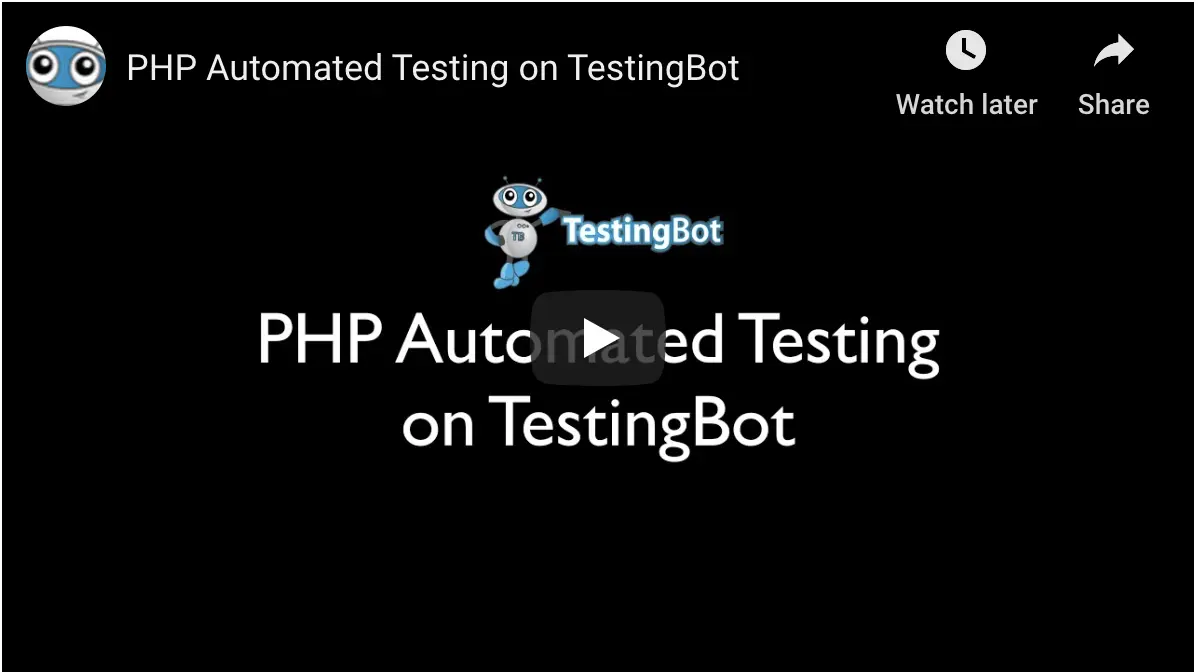
See our PHP example repository for a first example
on how to run PHP tests in parallel on TestingBot.
Installation
With TestingBot you can easily run your automated tests with any PHP test framework, here's a first example with php-webdriver:
Save the example code below in a file called sampletest.php
<?php
require_once('vendor/autoload.php');
use Facebook\WebDriver\Remote\RemoteWebDriver;
use Facebook\WebDriver\WebDriverBy;
$web_driver = RemoteWebDriver::create(
"https://api_key:api_secret@hub.testingbot.com/wd/hub",
array("platform"=>"WINDOWS", "browserName"=>"chrome", "version" => "latest", "name" => "First Test"), 120000
);
$web_driver->get("https://google.com");
$element = $web_driver->findElement(WebDriverBy::name("q"));
if($element) {
$element->sendKeys("TestingBot");
$element->submit();
}
print $web_driver->getTitle();
$web_driver->quit();
?>
You can now run this test:
Configuring capabilities
To run your existing tests on TestingBot, your tests will need to be configured to use the TestingBot remote machines. If the test was running on your local machine or network, you can simply change your existing test like this:
Before: After:Specify Browsers & Devices
To let TestingBot know on which browser/platform/device you want to run your test on, you need to specify the browsername, version, OS and other optional options in the capabilities field.
$web_driver = RemoteWebDriver::create(
"http://api_key:api_secret@hub.testingbot.com/wd/hub",
$caps, 120000);
To see how to do this, please select a combination of browser, version and platform in the drop-down menus below.
Testing Internal Websites
We've built TestingBot Tunnel, to provide you with a secure way to run tests against your staged/internal webapps.
Please see our TestingBot Tunnel documentation for more information about this easy to use tunneling solution.
The example below shows how to easily run a PHP WebDriver test with our Tunnel:
1. Download our tunnel and start the tunnel:
2. Adjust your test: instead of pointing to 'hub.testingbot.com/wd/hub'
like the example above - change it to point to your tunnel's IP address.
Assuming you run the tunnel on the same machine you run your tests, change to 'localhost:4445/wd/hub'
. localhost is the machine running the tunnel, 4445 is the default port of the tunnel.
This way your test will go securely through the tunnel to TestingBot and back:
<?php
require_once('vendor/autoload.php');
use Facebook\WebDriver\Remote\RemoteWebDriver;
use Facebook\WebDriver\WebDriverBy;
$web_driver = RemoteWebDriver::create(
"https://api_key:api_secret@localhost:4445/wd/hub",
array("platform"=>"WINDOWS", "browserName"=>"firefox", "version" => "latest", "name" => "First Test"), 120000
);
$web_driver->get("https://google.com");
$element = $web_driver->findElement(WebDriverBy::name("q"));
if($element) {
$element->sendKeys("TestingBot");
$element->submit();
}
print $web_driver->getTitle();
$web_driver->quit();
?>
Run tests in Parallel
Parallel Testing means running the same test, or multiple tests, simultaneously. This greatly reduces your total testing time.
You can run the same tests on all different browser configurations or run different tests all on the same browser configuration.
TestingBot has a large grid of machines and browsers, which means you can use our service to do efficient parallel testing. It is one of the key features we provide to greatly cut down on your total testing time.
To run tests in parallel, we recommend using Paratest, which makes it very easy to run multiple PHPUnit tests simultaneously.
The command above will run your tests in 8 seperate processes (8 tests at once).
Queuing
Every plan we provide comes with a limit of parallel tests.
If you exceed the number of parallel tests assigned to your account, TestingBot will queue the additional tests (for up to 6 minutes) and run the tests as soon as slots become available.
Mark tests as passed/failed
As TestingBot has no way to dermine whether your test passed or failed (it is determined by your business logic), we offer a way to send the test status back to TestingBot. This is useful if you want to see if a test succeeded or failed from the TestingBot member area.
You can use our PHP API client to report back test results.
Other PHP Framework examples
-
Behat
Behat is a BDD framework which runs on PHP.
Mink is used for its browser emulation and works nicely together with Behat. -
Codeception
Codeception is a BDD-styled PHP testing framework.
This testing framework offers good Selenium support. -
Laravel Dusk
Laravel Dusk provides an easy-to-use browser automation testing framework.
-
PHPUnit
PHPUnit is the most popular unit testing framework for PHP.
It comes with good Selenium WebDriver support and is easy to set up. -
SimpleTest
SimpleTest is a framework for unit testing, web site testing and mock objects for PHP.